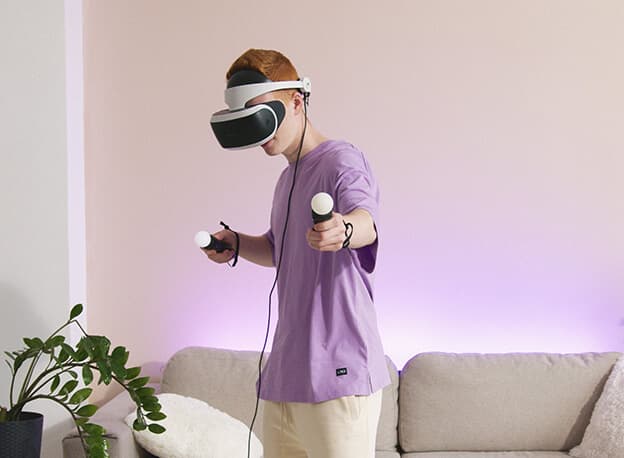
Blog components
Components associated with the blog/news section of the website.
Post preview card: Vertical
import Stack from 'react-bootstrap/Stack'
import BlogPostCard from '@/components/blog/blog-post-card'
export default function BlogPostCardVerticalDemo() {
return (
<Stack gap={4} style={{ maxWidth: 416 }}>
{/* Blog post preview card with category and date */}
<BlogPostCard
href="#"
image={{
src: '/img/blog/grid/v1/10.jpg',
width: 548,
height: 402,
alt: 'Image',
}}
title="Immersive worlds: A dive into the latest VR gear and experiences"
date="July 27, 2025"
category={{
label: 'Gaming',
href: '#',
}}
/>
{/* Blog post preview card with category, author and date */}
<BlogPostCard
href="#"
image={{
src: '/img/blog/grid/v1/09.jpg',
width: 548,
height: 402,
alt: 'Image',
}}
title="Feng shui your home: Interior design tips for positive energy"
date="June 27, 2025"
category={{
label: 'Interior design',
href: '#',
}}
author={{
name: 'Emily Davies',
href: '#',
}}
/>
</Stack>
)
}
Post preview card: Horizontal
How to find the best deals and make secure transactions online
This blog post will guide you through the dual objectives of snagging great bargains and protecting your financial...
import BlogPostCard from '@/components/blog/blog-post-card'
export default function BlogPostCardHorizontalDemo() {
return (
<BlogPostCard
horizontal
href="#"
image={{
src: '/img/blog/list/07.jpg',
width: 420,
height: 310,
alt: 'Image',
}}
title="How to find the best deals and make secure transactions online"
date="August 18, 2025"
category={{
label: 'Buying guides',
href: '#',
}}
excerpt="This blog post will guide you through the dual objectives of snagging great bargains and protecting your financial..."
/>
)
}
Vlog card
import VlogPostCard from '@/components/blog/vlog-post-card'
export default function VlogPostCardDemo() {
return (
<VlogPostCard
href="#"
image={{
src: '/img/blog/list/07.jpg',
width: 402,
height: 262,
alt: 'Image',
}}
title="A comprehensive review of the latest kitchen blenders on the market"
time="06:12"
style={{ maxWidth: 306 }}
/>
)
}
Featured post: Variant 1
Decorate your home in easy steps
Decorating your home can be a fun and creative process that transforms your living space.
Learn moreimport Image from 'next/image'
import Link from 'next/link'
import Row from 'react-bootstrap/Row'
import Col from 'react-bootstrap/Col'
import Nav from 'react-bootstrap/Nav'
import NavLink from 'react-bootstrap/NavLink'
import Lightbox from '@/components/lightbox'
export default function FeaturedPostVariantOneDemo() {
return (
<Row xs={1} md={2} className="g-0 overflow-hidden rounded-5">
<Col className="position-relative">
<div className="ratio ratio-4x3" />
<Image
src="/img/blog/grid/v2/video01.jpg"
fill
sizes="(max-width: 768px) 100vw, 50vw"
className="object-fit-cover"
alt="Image"
/>
<div className="position-absolute start-0 bottom-0 d-flex align-items-end w-100 h-100 z-2 p-4">
<Lightbox
href="https://www.youtube.com/watch?v=X7lCwxswYnE"
gallery="video"
className="btn btn-lg btn-light rounded-pill m-md-2"
>
<i className="ci-play fs-lg ms-n1 me-2"/>
Play
</Lightbox>
</div>
</Col>
<Col className="bg-dark py-5 px-4 px-xl-5" data-bs-theme="dark">
<div className="py-md-2 py-xl-4 px-md-3 px-xl-4">
<Nav className="mb-3">
<NavLink as={Link} href="#" className="text-body fs-xs text-uppercase p-0">
Home decoration
</NavLink>
</Nav>
<h2>Decorate your home in easy steps</h2>
<p className="text-body pb-sm-2 pb-lg-0 mb-4 mb-lg-5">
Decorating your home can be a fun and creative process that transforms your living space.
</p>
<Link href="#" className="btn btn-lg btn-outline-light rounded-pill">
Learn more
</Link>
</div>
</Col>
</Row>
)
}
Featured post: Variant 2
The role of philanthropy in building a better world
Charitable contributions are a vital aspect of building a better world. These contributions come in various forms, including monetary donations...
Learn moreimport Image from 'next/image'
import Link from 'next/link'
import Row from 'react-bootstrap/Row'
import Col from 'react-bootstrap/Col'
import Lightbox from '@/components/lightbox'
export default function FeaturedPostVariantTwoDemo() {
return (
<Row xs={1} md={2} className="g-4">
<Col>
<div className="position-relative h-100">
<div className="ratio ratio-16x9" />
<Image
src="/img/about/v1/video-cover.jpg"
fill
sizes="(max-width: 768px) 100vw, 50vw"
className="object-fit-cover rounded-5"
alt="Image"
/>
<div className="position-absolute start-0 bottom-0 d-flex align-items-end w-100 h-100 z-2 p-4">
<Lightbox
href="https://www.youtube.com/watch?v=Sqqj_14wBxU"
gallery="video"
className="btn btn-lg btn-light rounded-pill m-md-2"
>
<i className="ci-play fs-lg ms-n1 me-2"/>
Play
</Lightbox>
</div>
</div>
</Col>
<Col>
<div className="bg-body-tertiary rounded-5 py-5 px-4 px-sm-5">
<div className="py-md-3 py-lg-4 py-xl-5 px-lg-4 px-xl-5">
<h2 className="h3 pb-sm-1 pb-lg-2">The role of philanthropy in building a better world</h2>
<p className="pb-sm-2 pb-lg-0 mb-4 mb-lg-5">
Charitable contributions are a vital aspect of building a better world. These contributions come in
various forms, including monetary donations...
</p>
<Link href="#" className="btn btn-lg btn-outline-dark">
Learn more
</Link>
</div>
</div>
</Col>
</Row>
)
}
Recipe card
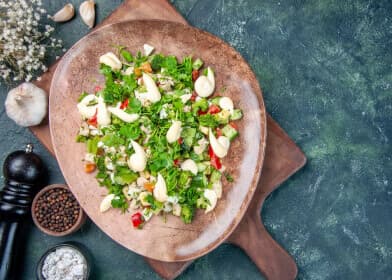
Garden salad with a mix of lettuce, cucumber and tomato
30 min
Easy
4 por
import RecipeCard from '@/components/blog/recipe-card'
export default function BlogPostNavigationDemo() {
return (
<RecipeCard
href="#"
image={{ src: '/img/home/grocery/recipes/01.jpg', alt: 'Image' }}
title="Garden salad with a mix of lettuce, cucumber and tomato"
timeToCook="30 min"
difficulty="Easy"
servings="4 por"
/>
)
}