Card
Flexible and extensible content container.
Basic example
Card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Go somewhereimport Card from 'react-bootstrap/Card'
import CardTitle from 'react-bootstrap/CardTitle'
import CardText from 'react-bootstrap/CardText'
import Button from 'react-bootstrap/Button'
export default function CardBasicDemo() {
return (
<Card body style={{ maxWidth: 350 }}>
<CardTitle as="h5">Card title</CardTitle>
<CardText>
Some quick example text to build on the card title and make up the bulk of the card's content.
</CardText>
<Button href="#">Go somewhere</Button>
</Card>
)
}
Alternative card style
Card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Go somewhereCard title
Some quick example text to build on the card title and make up the bulk of the card's content.
Go somewhereimport Card from 'react-bootstrap/Card'
import CardTitle from 'react-bootstrap/CardTitle'
import CardText from 'react-bootstrap/CardText'
import Button from 'react-bootstrap/Button'
export default function CardAltStyleDemo() {
return (
<div className="d-flex flex-column flex-sm-row gap-4">
<Card body className="bg-body-tertiary border-0" style={{ maxWidth: 350 }}>
<CardTitle as="h5">Card title</CardTitle>
<CardText>
Some quick example text to build on the card title and make up the bulk of the card's content.
</CardText>
<Button href="#">Go somewhere</Button>
</Card>
<Card body className="border-0 shadow" style={{ maxWidth: 350 }}>
<CardTitle as="h5">Card title</CardTitle>
<CardText>
Some quick example text to build on the card title and make up the bulk of the card's content.
</CardText>
<Button href="#">Go somewhere</Button>
</Card>
</div>
)
}
Image caps
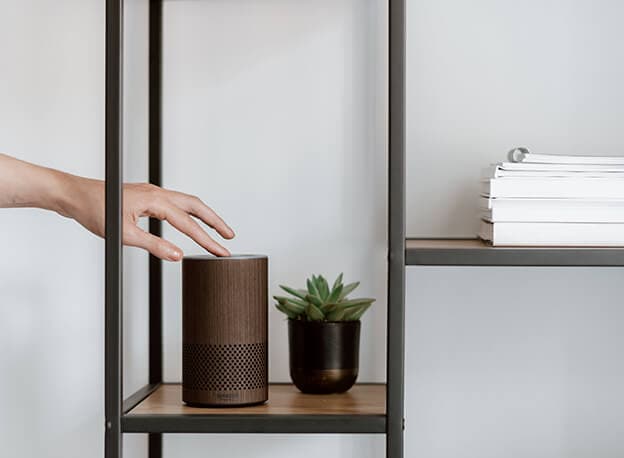
Card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Go somewhereCard title
Some quick example text to build on the card title and make up the bulk of the card's content.
Go somewhere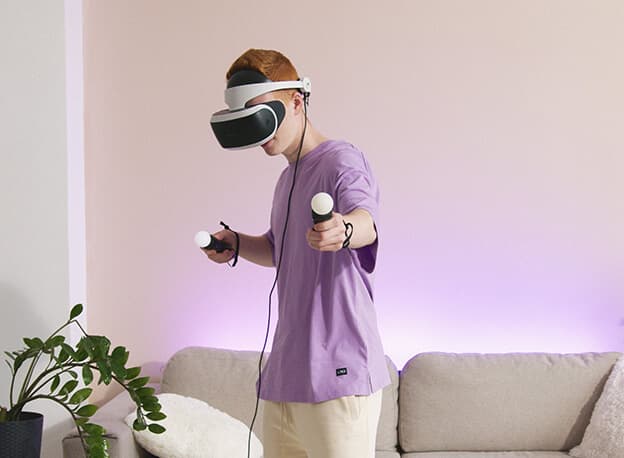
import Image from 'next/image'
import Card from 'react-bootstrap/Card'
import CardTitle from 'react-bootstrap/CardTitle'
import CardText from 'react-bootstrap/CardText'
import Button from 'react-bootstrap/Button'
export default function CardImageCapsDemo() {
return (
<div className="d-flex flex-column flex-sm-row gap-4">
<Card style={{ maxWidth: 350 }}>
<CardImg
as={Image}
variant="top"
src="/img/blog/grid/v1/07.jpg"
width={624}
height={458}
className="bg-body-tertiary"
alt="Card image"
/>
<CardBody>
<CardTitle as="h5">Card title</CardTitle>
<CardText>
Some quick example text to build on the card title and make up the bulk of the card's content.
</CardText>
<Button href="#">Go somewhere</Button>
</CardBody>
</Card>
<Card style={{ maxWidth: 350 }}>
<CardBody>
<CardTitle as="h5">Card title</CardTitle>
<CardText>
Some quick example text to build on the card title and make up the bulk of the card's content.
</CardText>
<Button href="#">Go somewhere</Button>
</CardBody>
<CardImg
as={Image}
variant="bottom"
src="/img/blog/grid/v1/10.jpg"
width={624}
height={458}
className="bg-body-tertiary"
alt="Card image"
/>
</Card>
</div>
)
}
Image hover effects
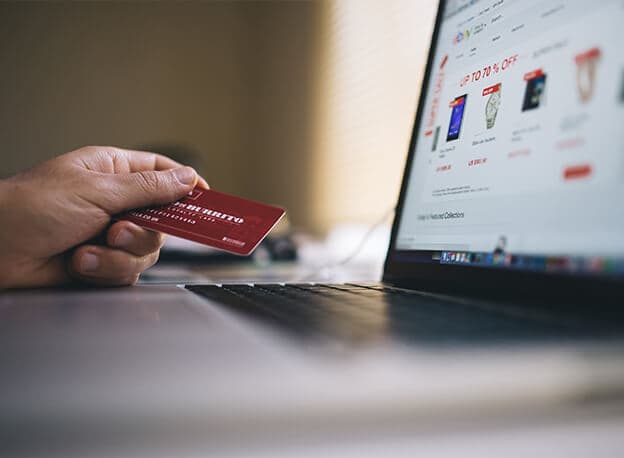
Card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Go somewhere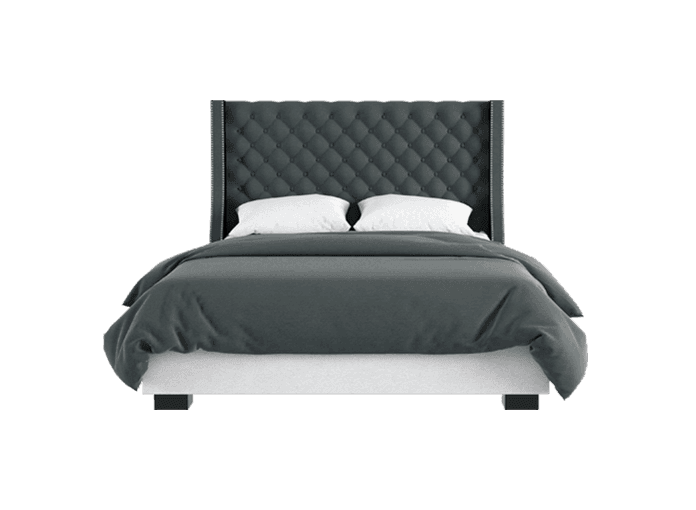
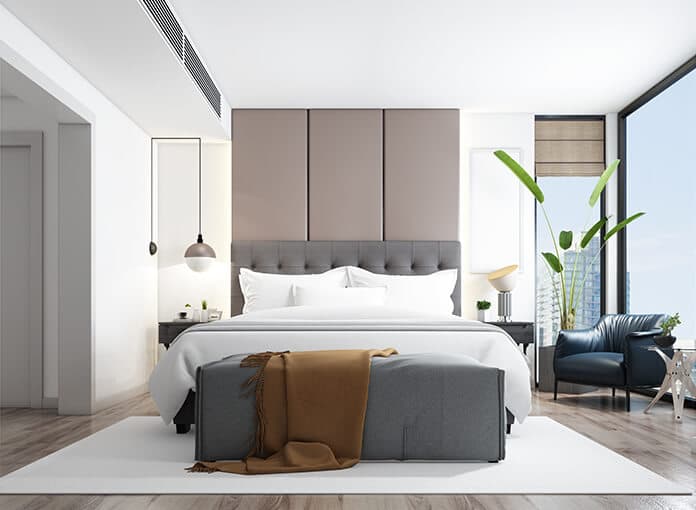
Card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Go somewhereimport Image from 'next/image'
import ImageSwapOnHover from '@/components/image-swap-on-hover'
import Card from 'react-bootstrap/Card'
import CardBody from 'react-bootstrap/CardBody'
import CardTitle from 'react-bootstrap/CardTitle'
import CardText from 'react-bootstrap/CardText'
import Button from 'react-bootstrap/Button'
export default function CardImageHoverDemo() {
return (
<div className="d-flex flex-column flex-sm-row gap-4">
<Card style={{ maxWidth: 350 }}>
<div className="hover-effect-scale hover-effect-opacity card-img-top position-relative overflow-hidden">
<span className="hover-effect-target position-absolute top-0 start-0 w-100 h-100 bg-black bg-opacity-25 opacity-0 z-1"></span>
<div className="hover-effect-target d-flex position-absolute top-0 start-0 w-100 h-100 align-items-center justify-content-center z-2 opacity-0">
<div className="d-flex align-items-center gap-3 fs-sm bg-dark bg-opacity-50 text-white rounded-pill py-2 px-3">
<span className="d-flex align-items-center fw-medium">
<i className="ci-heart fs-base me-1"/>
12
</span>
<span className="d-flex align-items-center fw-medium">
<i className="ci-message-square fs-base me-1"/>8
</span>
</div>
</div>
<Image
src="/img/blog/grid/v1/08.jpg"
width={624}
height={458}
className="hover-effect-target"
alt="Card image"
/>
</div>
<CardBody>
<CardTitle as="h5">Card title</CardTitle>
<CardText>
Some quick example text to build on the card title and make up the bulk of the card's content.
</CardText>
<Button href="#">Go somewhere</Button>
</CardBody>
</Card>
<Card style={{ maxWidth: 350 }}>
<ImageSwapOnHover
imageSwapFrom={{
src: '/img/docs/card/img.png',
width: 624,
height: 458,
alt: 'Image idle',
}}
imageSwapTo={{
src: '/img/docs/card/img-hover.jpg',
width: 624,
height: 458,
alt: 'Image hover',
}}
className="card-img-top"
/>
<CardBody>
<CardTitle as="h5">Card title</CardTitle>
<CardText>
Some quick example text to build on the card title and make up the bulk of the card's content.
</CardText>
<Button href="#">Go somewhere</Button>
</CardBody>
</Card>
</div>
)
}
Horizontal layout
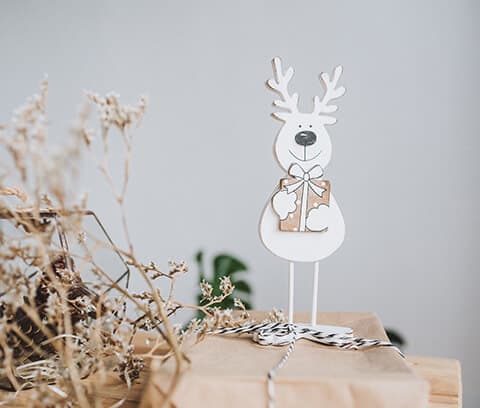
Card title
This is a wider card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.
Go somewhere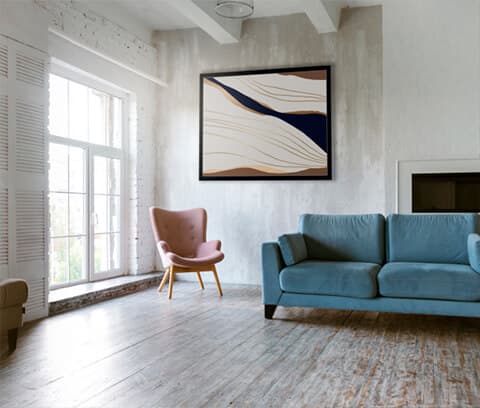
Card title
This is a wider card with supporting text below as a natural lead-in to additional content. This content is a little bit longer.
Go somewhereimport Image from 'next/image'
import Card from 'react-bootstrap/Card'
import CardBody from 'react-bootstrap/CardBody'
import CardTitle from 'react-bootstrap/CardTitle'
import CardText from 'react-bootstrap/CardText'
import Row from 'react-bootstrap/Row'
import Col from 'react-bootstrap/Col'
import Button from 'react-bootstrap/Button'
export default function CardHorizontalDemo() {
return (
<>
<Card className="overflow-hidden mb-4">
<Row className="g-0">
<Col sm={4} className="position-relative bg-body-tertiary" style={{ minHeight: 220 }}>
<Image src="/img/blog/grid/v2/09.jpg" fill={true} className="object-fit-cover" alt="Card image" />
</Col>
<Col sm={8}>
<CardBody>
<CardTitle as="h5">Card title</CardTitle>
<CardText>
This is a wider card with supporting text below as a natural lead-in to additional content. This
content is a little bit longer.
</CardText>
<Button href="#">Go somewhere</Button>
</CardBody>
</Col>
</Row>
</Card>
<Card className="overflow-hidden mb-4">
<Row className="g-0">
<Col sm={4} className="position-relative order-sm-2 bg-body-tertiary" style={{ minHeight: 220 }}>
<Image src="/img/blog/grid/v2/11.jpg" fill={true} className="object-fit-cover" alt="Card image" />
</Col>
<Col sm={8} className="order-sm-1">
<CardBody>
<CardTitle as="h5">Card title</CardTitle>
<CardText>
This is a wider card with supporting text below as a natural lead-in to additional content. This
content is a little bit longer.
</CardText>
<Button href="#">Go somewhere</Button>
</CardBody>
</Col>
</Row>
</Card>
</>
)
}
Text alignment
import Row from 'react-bootstrap/Row'
import Col from 'react-bootstrap/Col'
import Card from 'react-bootstrap/Card'
import CardTitle from 'react-bootstrap/CardTitle'
import CardText from 'react-bootstrap/CardText'
import Button from 'react-bootstrap/Button'
export default function CardTextAlignmentDemo() {
return (
<Row xs={1} md={3} className="g-4">
{['start', 'center', 'end'].map((alignment) => (
<Col key={alignment}>
<Card body className={`text-${alignment}`}>
<CardTitle as="h5">Card title</CardTitle>
<CardText>With supporting text below as a natural lead-in to additional content.</CardText>
<Button href="#">Go somewhere</Button>
</Card>
</Col>
))}
</Row>
)
}
List group inside card
Card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Card title
Some quick example text to build on the card title and make up the bulk of the card's content.
import Row from 'react-bootstrap/Row'
import Col from 'react-bootstrap/Col'
import Card from 'react-bootstrap/Card'
import CardBody from 'react-bootstrap/CardBody'
import CardTitle from 'react-bootstrap/CardTitle'
import CardText from 'react-bootstrap/CardText'
import Button from 'react-bootstrap/Button'
import ListGroup from 'react-bootstrap/ListGroup'
import ListGroupItem from 'react-bootstrap/ListGroupItem'
export default function CardListGroupDemo() {
return (
<Row xs={1} md={w} className="g-4">
<Col>
<Card>
<CardBody>
<CardTitle as="h5">Card title</CardTitle>
<CardText>
Some quick example text to build on the card title and make up the bulk of the card's content.
</CardText>
</CardBody>
<ListGroup className="list-group-flush fs-base">
<ListGroupItem>Lorem ipsum dolor sit</ListGroupItem>
<ListGroupItem>Cras justo odio</ListGroupItem>
<ListGroupItem>Dapibus ac facilisis in</ListGroupItem>
<ListGroupItem>Vestibulum at eros</ListGroupItem>
</ListGroup>
<CardBody>
<Button href="#">Go somewhere</Button>
</CardBody>
</Card>
</Col>
<Col>
<Card>
<CardBody>
<CardTitle as="h5">Card title</CardTitle>
<CardText>
Some quick example text to build on the card title and make up the bulk of the card's content.
</CardText>
</CardBody>
<ListGroup className="list-group-flush fs-base">
<ListGroupItem action href="#link1">
Lorem ipsum dolor sit
</ListGroupItem>
<ListGroupItem action href="#link2">
Cras justo odio
</ListGroupItem>
<ListGroupItem action href="#link3">
Dapibus ac facilisis in
</ListGroupItem>
<ListGroupItem action href="#link4">
Vestibulum at eros
</ListGroupItem>
</ListGroup>
<CardBody>
<Button href="#">Go somewhere</Button>
</CardBody>
</Card>
</Col>
</Row>
)
}
Card styles: Solid background
Primary card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Secondary card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Success card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Danger card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Warning card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Info card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Body secondary card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Dark card title
Some quick example text to build on the card title and make up the bulk of the card's content.
import Row from 'react-bootstrap/Row'
import Col from 'react-bootstrap/Col'
import Card from 'react-bootstrap/Card'
import CardBody from 'react-bootstrap/CardBody'
import CardHeader from 'react-bootstrap/CardHeader'
import CardTitle from 'react-bootstrap/CardTitle'
import CardText from 'react-bootstrap/CardText'
export default function CardSolidBgDemo() {
return (
<Row xs={1} sm={2} className="g-4">
{['primary', 'secondary', 'success', 'danger', 'warning', 'info', 'body secondary', 'dark'].map((color) => (
<Col key={color}>
<Card className={color === 'body secondary' ? 'bg-body-secondary' : `text-bg-${color}`}>
<CardHeader>Header</CardHeader>
<CardBody>
<CardTitle as="h5" className={color !== 'body secondary' ? 'text-white' : ''}>
{color.charAt(0).toUpperCase() + color.slice(1).toLowerCase()} card title
</CardTitle>
<CardText>
Some quick example text to build on the card title and make up the bulk of the card's content.
</CardText>
</CardBody>
</Card>
</Col>
))}
</Row>
)
}
Card styles: Subtle background
Primary card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Secondary card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Success card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Danger card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Warning card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Info card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Dark card title
Some quick example text to build on the card title and make up the bulk of the card's content.
import Row from 'react-bootstrap/Row'
import Col from 'react-bootstrap/Col'
import Card from 'react-bootstrap/Card'
import CardBody from 'react-bootstrap/CardBody'
import CardHeader from 'react-bootstrap/CardHeader'
import CardTitle from 'react-bootstrap/CardTitle'
import CardText from 'react-bootstrap/CardText'
export default function CardSubtleBgDemo() {
return (
<Row xs={1} sm={2} className="g-4">
{['primary', 'secondary', 'success', 'danger', 'warning', 'info', 'dark'].map((color) => (
<Col key={color}>
<Card className={`bg-${color}-subtle border-${color}-subtle`}>
<CardHeader className={`border-${color}-subtle`}>Header</CardHeader>
<CardBody>
<CardTitle as="h5">
{color.charAt(0).toUpperCase() + color.slice(1).toLowerCase()} card title
</CardTitle>
<CardText>
Some quick example text to build on the card title and make up the bulk of the card's content.
</CardText>
</CardBody>
</Card>
</Col>
))}
</Row>
)
}
Card styles: Border and text color
Primary card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Secondary card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Success card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Danger card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Warning card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Info card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Dark card title
Some quick example text to build on the card title and make up the bulk of the card's content.
import Row from 'react-bootstrap/Row'
import Col from 'react-bootstrap/Col'
import Card from 'react-bootstrap/Card'
import CardBody from 'react-bootstrap/CardBody'
import CardHeader from 'react-bootstrap/CardHeader'
import CardTitle from 'react-bootstrap/CardTitle'
import CardText from 'react-bootstrap/CardText'
export default function CardBorderColorDemo() {
return (
<Row xs={1} sm={2} className="g-4">
{['primary', 'secondary', 'success', 'danger', 'warning', 'info', 'dark'].map((color) => (
<Col key={color}>
<Card className={`text-${color === 'dark' ? 'body-emphasis' : color} border-${color}`}>
<CardHeader className={`bg-${color}-subtle border-${color}`}>Header</CardHeader>
<CardBody>
<CardTitle as="h5" className={`text-${color === 'dark' ? 'body-emphasis' : color}`}>
{color.charAt(0).toUpperCase() + color.slice(1).toLowerCase()} card title
</CardTitle>
<CardText>
Some quick example text to build on the card title and make up the bulk of the card's content.
</CardText>
</CardBody>
</Card>
</Col>
))}
</Row>
)
}
Layout: Card group
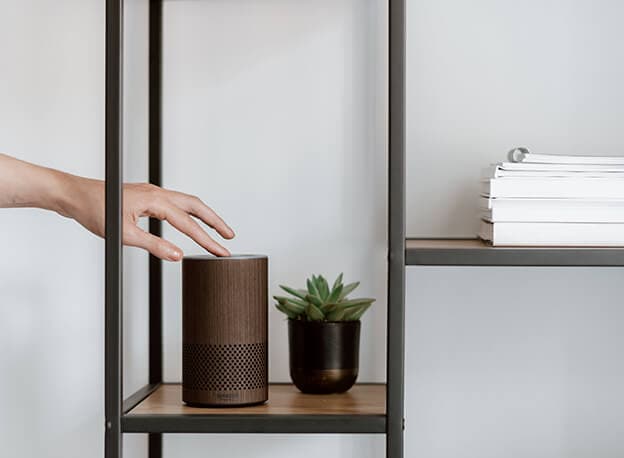
Card title
This is a wider card with supporting text below as a natural lead-in to additional content. This card has even longer content than the first to show that equal height action.
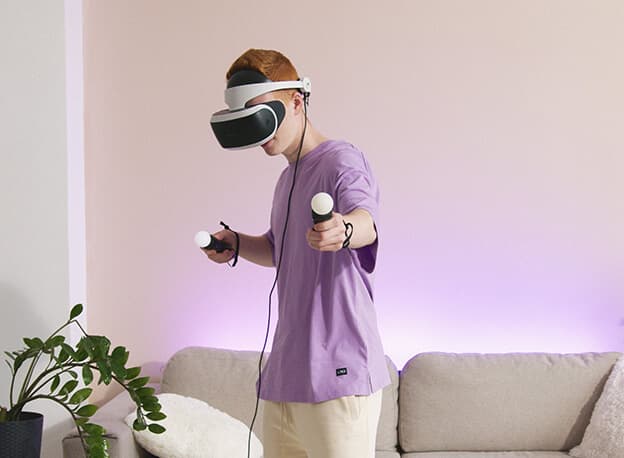
Card title
This card has supporting text below as a natural lead-in to additional content.
import Image from 'next/image'
import CardGroup from 'react-bootstrap/CardGroup'
import Card from 'react-bootstrap/Card'
import CardImg from 'react-bootstrap/CardImg'
import CardBody from 'react-bootstrap/CardBody'
import CardFooter from 'react-bootstrap/CardFooter'
import CardTitle from 'react-bootstrap/CardTitle'
import CardText from 'react-bootstrap/CardText'
export default function CardGroupDemo() {
return (
<CardGroup>
<Card>
<CardImg
as={Image}
variant="top"
src="/img/blog/grid/v1/07.jpg"
width={624}
height={458}
className="bg-body-tertiary"
alt="Card image"
/>
<CardBody>
<CardTitle as="h5">Card title</CardTitle>
<CardText>
This is a wider card with supporting text below as a natural lead-in to additional content. This card
has even longer content than the first to show that equal height action.
</CardText>
</CardBody>
<CardFooter className="text-body-tertiary bg-transparent border-0 fs-sm pt-0 pb-4">
Last updated 3 mins ago
</CardFooter>
</Card>
<Card>
<CardImg
as={Image}
variant="top"
src="/img/blog/grid/v1/10.jpg"
width={624}
height={458}
className="bg-body-tertiary"
alt="Card image"
/>
<CardBody>
<CardTitle as="h5">Card title</CardTitle>
<CardText>This card has supporting text below as a natural lead-in to additional content.</CardText>
</CardBody>
<CardFooter className="text-body-tertiary bg-transparent border-0 fs-sm pt-0 pb-4">
Last updated 5 mins ago
</CardFooter>
</Card>
</CardGroup>
)
}
Layout: Card grid
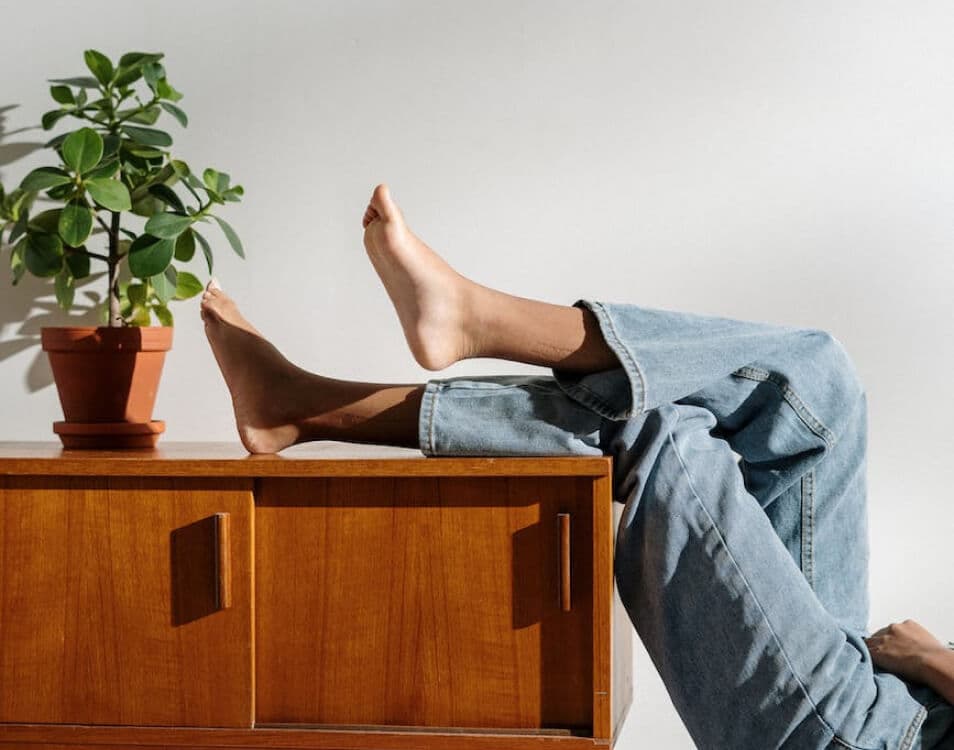
Card title
Some quick example text to build on the card title.
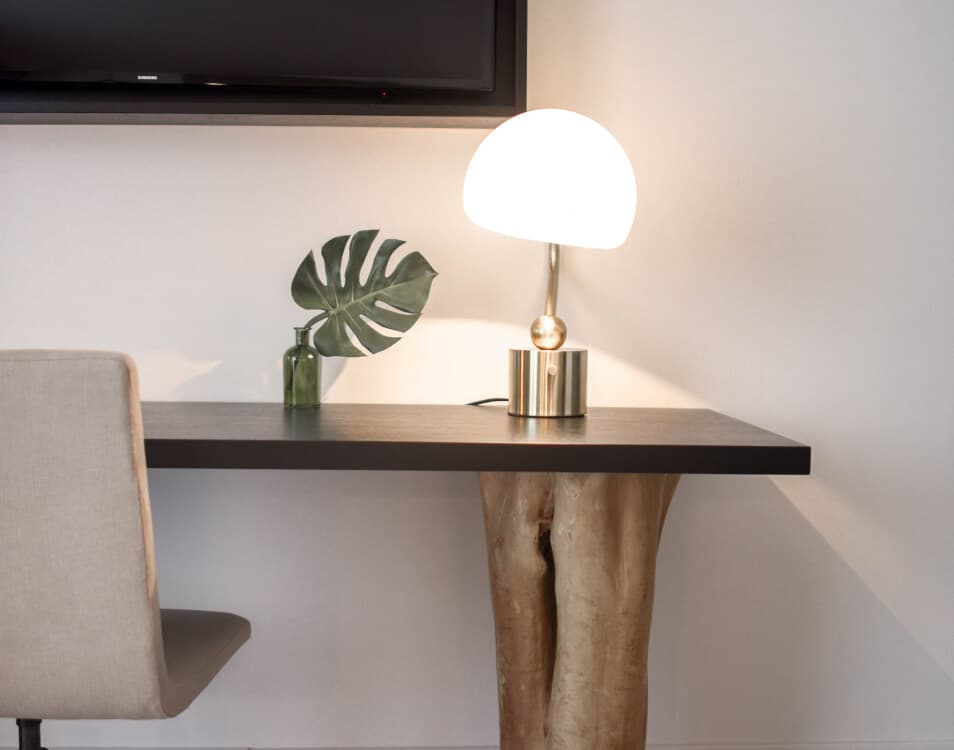
Card title
Some quick example text to build on the card title.
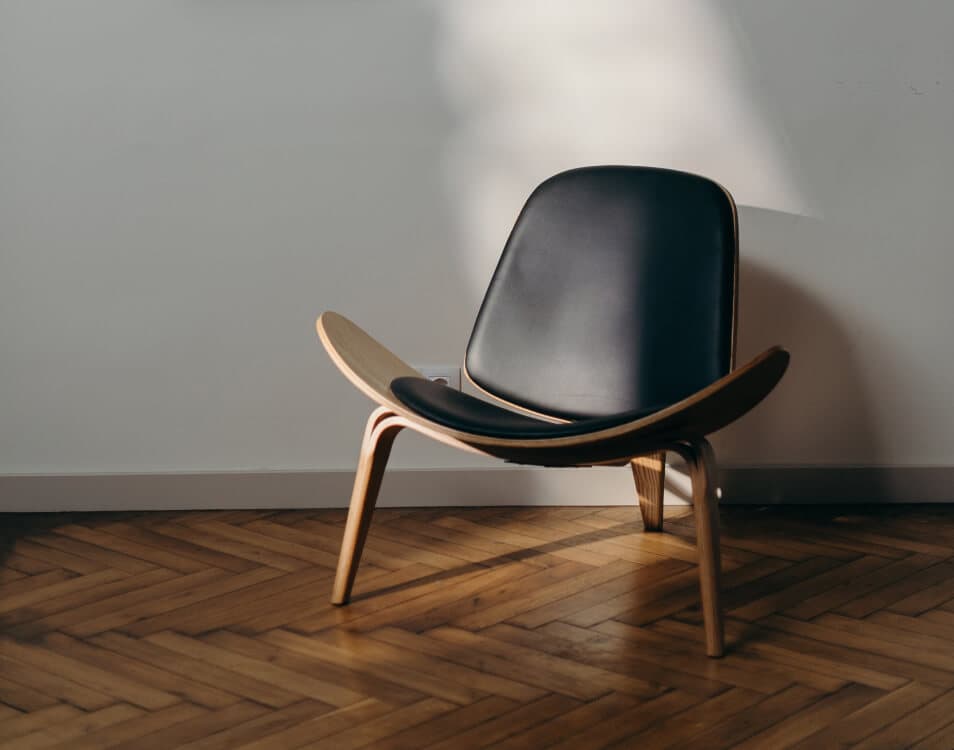
Card title
Some quick example text to build on the card title.
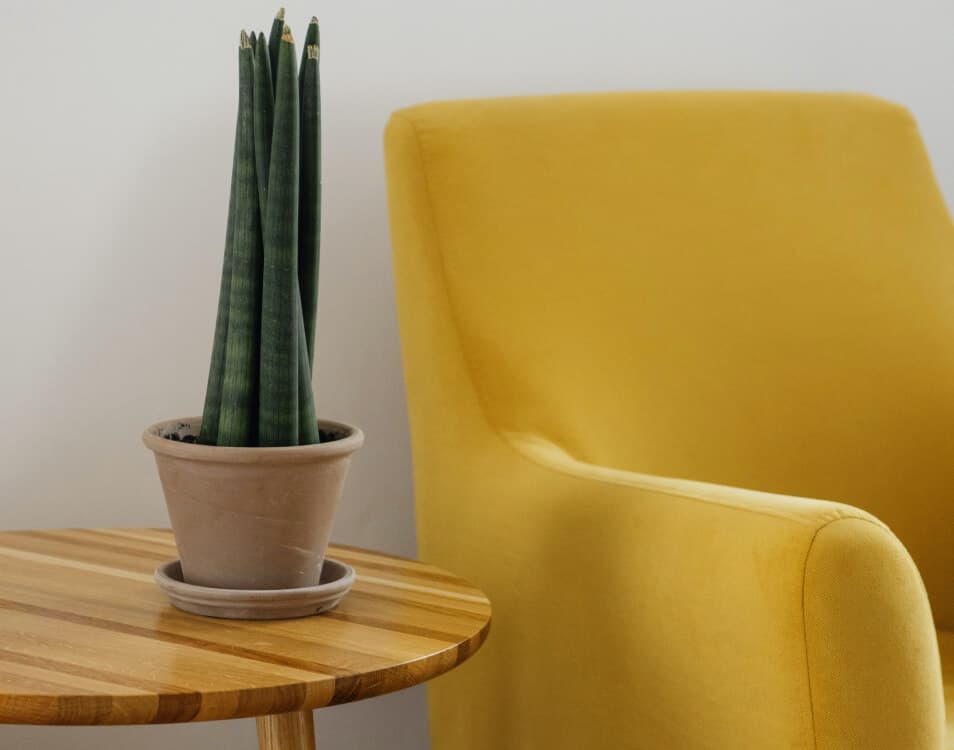
Card title
Some quick example text to build on the card title.
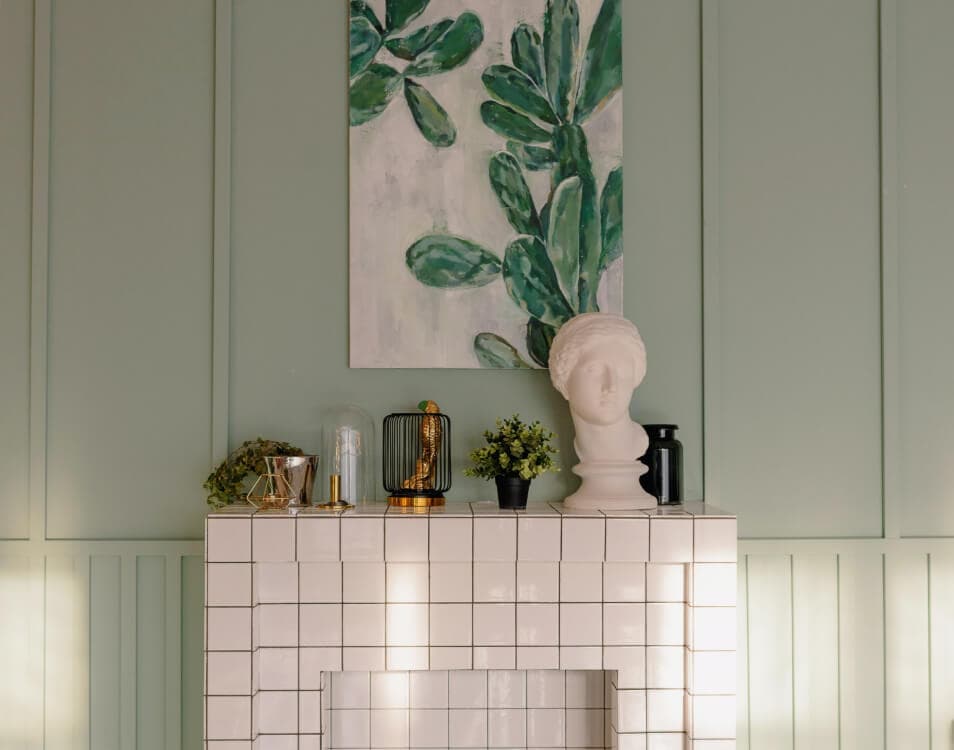
Card title
Some quick example text to build on the card title.
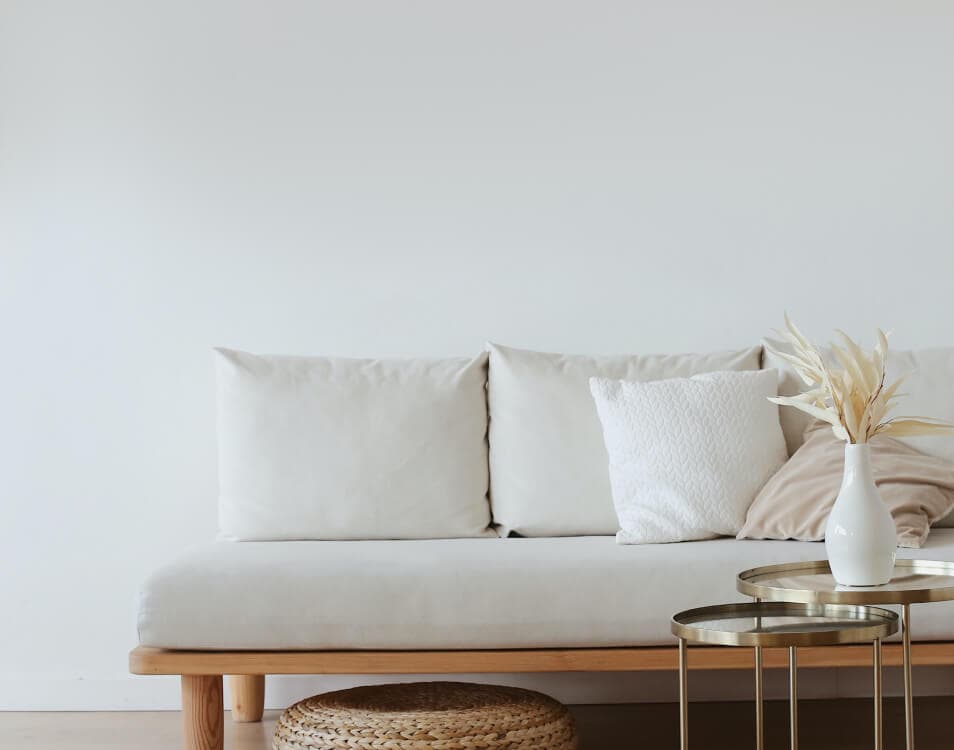
Card title
Some quick example text to build on the card title.
import Image from 'next/image'
import Row from 'react-bootstrap/Row'
import Col from 'react-bootstrap/Col'
import Card from 'react-bootstrap/Card'
import CardImg from 'react-bootstrap/CardImg'
import CardBody from 'react-bootstrap/CardBody'
import CardTitle from 'react-bootstrap/CardTitle'
import CardText from 'react-bootstrap/CardText'
export default function CardGridDemo() {
return (
<Row xs={1} sm={2} md={3} className="g-4">
{[
'/img/blog/grid/v2/01.jpg',
'/img/blog/grid/v2/02.jpg',
'/img/blog/grid/v2/03.jpg',
'/img/blog/grid/v2/04.jpg',
'/img/blog/grid/v2/05.jpg',
'/img/blog/grid/v2/06.jpg',
].map((image, index) => (
<Col key={index}>
<Card>
<CardImg
as={Image}
variant="top"
src={image}
width={636}
height={466}
className="bg-body-tertiary"
alt="Card image"
/>
<CardBody>
<CardTitle as="h5">Card title</CardTitle>
<CardText>Some quick example text to build on the card title.</CardText>
</CardBody>
</Card>
</Col>
))}
</Row>
)
}