Image zoom
JavaScript based component that adds "zoom on hover" functionality to images.
The ImageZoom component is a React wrapper for the Drift.js plugin. This allows you to configure most common Drift.js options directly through props.
Available props:
src
- The source URL of the main image.zoomSrc
- The source URL of the zoomed-in version of the image.width
- The width of the image in pixels.height
- The height of the image in pixels.alt
- Alternative text for the image, used for accessibility and SEO.paneContainerId
- The ID of the container element where the zoom pane will be rendered. Optional.inlinePane={375}
- Will switch to inline when zoom panewindowWidth <= inlinePane
. Set this value to big number (ex. 5000) for the ZoomPane to be always inline.hoverDelay
- Delay in milliseconds before the zoom pane appears when hovering over the image.touchDelay
- Delay in milliseconds before the zoom pane appears on touch events.touchDisable
- If true, disables the zoom functionality on touch devices.imageClassName
- Custom CSS class to apply to the image element.onShow={() => console.log('ZoomPane is shown')}
- Callback function triggered when the zoom pane is shown.onHide={() => console.log('ZoomPane is hidden')}
- Callback function triggered when the zoom pane is hidden.
External zoom pane
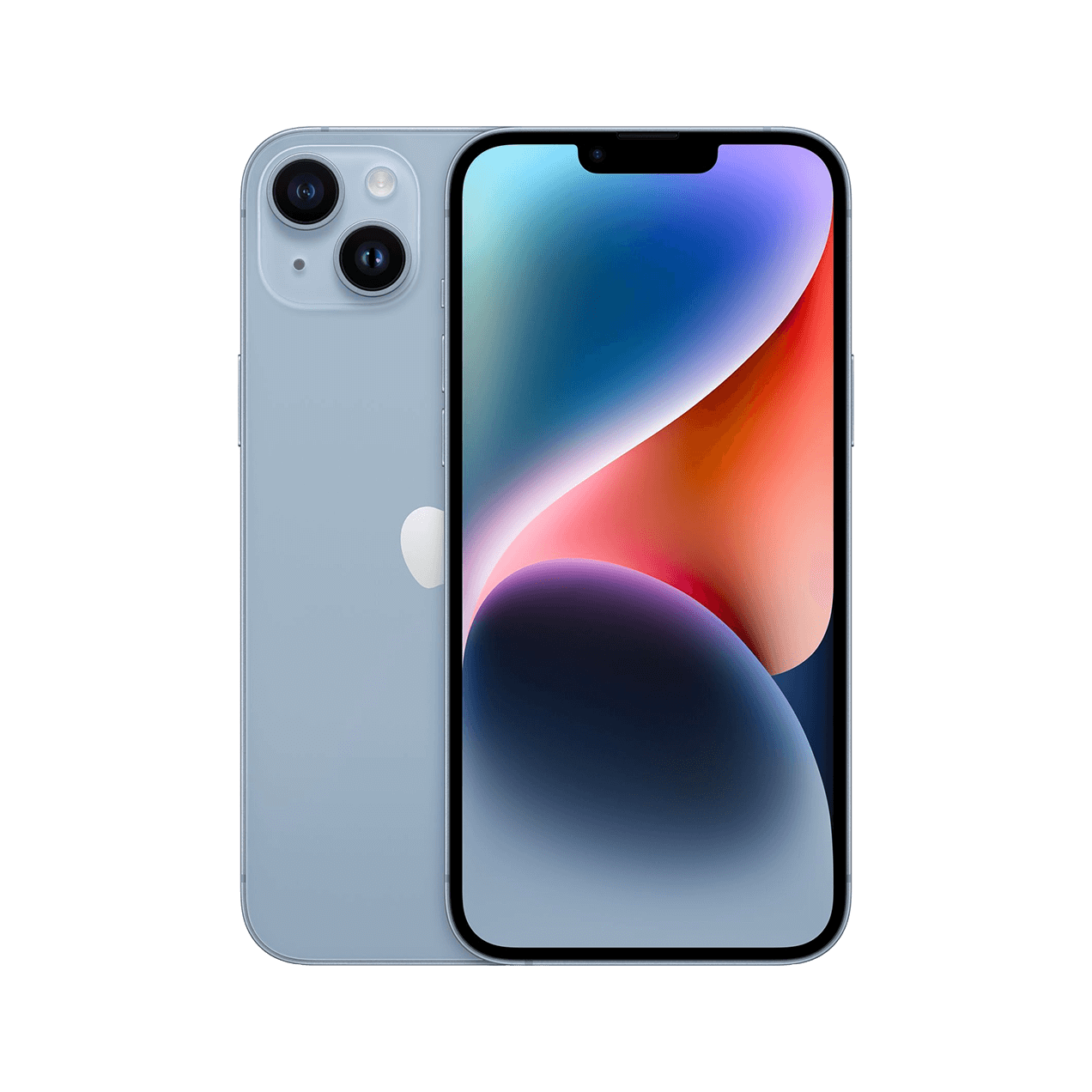
68 reviews
Apple iPhone 14 Plus 128GB
- Model: 128GB
- Color: Gray blue
- Capacity: 128GB
$940.00
import Row from 'react-bootstrap/Row'
import Col from 'react-bootstrap/Col'
import Button from 'react-bootstrap/Button'
import ImageZoom from '@/components/image/image-zoom'
import StarRating from '@/components/reviews/star-rating'
export default function ImageZoomExternalPaneDemo() {
return (
<Row className="align-items-md-center justify-content-center g-0">
<Col xs={10} sm={6}>
<ImageZoom
src="/img/shop/electronics/product/gallery/01.png"
zoomSrc="/img/shop/electronics/product/gallery/01.png"
paneContainerId="zoomPane"
hoverDelay={300}
width={636}
height={636}
alt="Image"
/>
</Col>
<Col sm={6} lg={5}>
<div className="position-relative p-2" id="zoomPane">
<div className="d-none d-md-flex align-items-center gap-2 text-decoration-none mb-3">
<StarRating rating={4.5} className="fs-sm" />
<span className="text-body-tertiary fs-xs">68 reviews</span>
</div>
<h2 className="h4">Apple iPhone 14 Plus 128GB</h2>
<ul className="list-unstyled">
<li className="form-label fw-semibold m-0">
Model:{' '}
<span className="text-body fw-normal" id="colorOption">
128GB
</span>
</li>
<li className="form-label fw-semibold m-0">
Color:{' '}
<span className="text-body fw-normal" id="colorOption">
Gray blue
</span>
</li>
<li className="form-label fw-semibold m-0">
Capacity:{' '}
<span className="text-body fw-normal" id="colorOption">
128GB
</span>
</li>
</ul>
<div className="h4 mb-4">$940.00</div>
<Button size="lg" className="animate-slide-end order-sm-2 order-md-4 order-lg-2">
<i className="ci-shopping-cart fs-lg animate-target me-2"/>
Add to cart
</Button>
</div>
</Col>
</Row>
)
}
Inline zoom pane
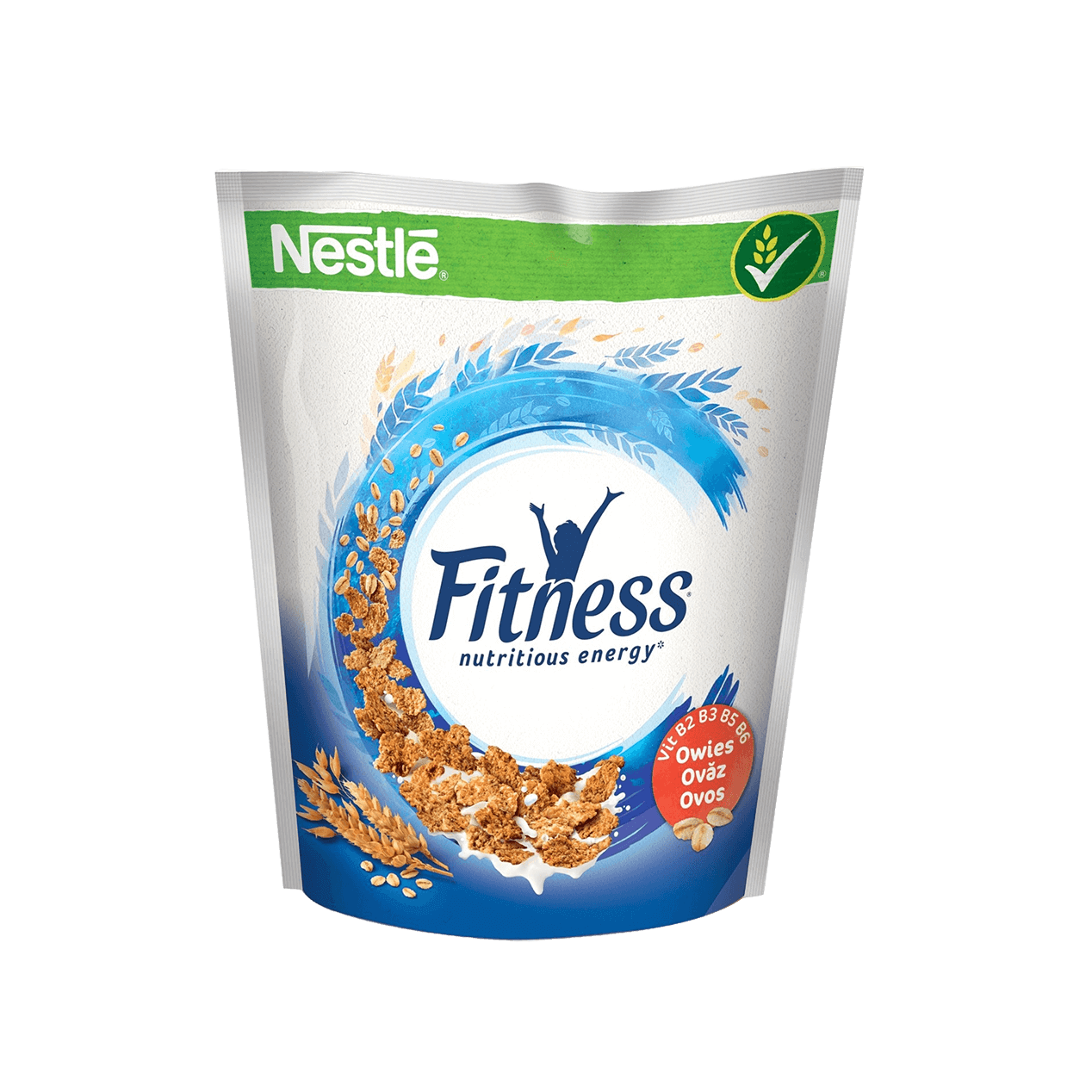
import ImageZoom from '@/components/image/image-zoom'
export default function ImageZoomInlinePaneDemo() {
return (
<ImageZoom
src="/img/shop/grocery/product/02.png"
zoomSrc="/img/shop/grocery/product/02.png"
inlinePane={5000}
hoverDelay={300}
width={634}
height={634}
alt="Image"
style={{ maxWidth: 540 }}
/>
)
}