Lightbox (Gallery)
JavaScript-based lightbox component designed to display various types of media.
The Lightbox component is a React wrapper for the Glightbox plugin.
Available props:
gallery={string}
- The identifier for the lightbox gallery. This value is used to group items in the same gallery.href={string}
- The URL of the image or video to be displayed in the lightbox.title={string}
- An optional title for the lightbox slide. This will appear as the caption.description={string}
- An optional description for the lightbox slide. This will appear under the title as additional text.fullscreen={boolean}
- Determines if the lightbox should display the slide in fullscreen mode. Default isfalse
.children
- The content to render as the clickable element that opens the lightbox.
Media: Image
import Image from 'next/image'
import Lightbox from '@/components/lightbox'
export default function LightboxImageDemo() {
return (
<Lightbox
href="/img/blog/grid/v2/07.jpg"
gallery="image"
title="Image caption"
description="Description goes here."
className="hover-effect-opacity d-flex rounded-4 overflow-hidden"
style={{ maxWidth: 480 }}
>
<div className="hover-effect-target position-absolute d-flex flex-column align-items-center top-0 start-0 w-100 h-100 z-2 text-white opacity-0 p-4">
<i className="ci-zoom-in fs-2 position-absolute top-50 translate-middle-y"/>
<span className="fs-sm fw-medium text-center mt-auto">Image caption</span>
</div>
<span className="hover-effect-target position-absolute top-0 start-0 w-100 h-100 bg-black bg-opacity-25 opacity-0 z-1"></span>
<Image src="/img/blog/grid/v2/07.jpg" width={600} height={440} alt="Image" />
</Lightbox>
)
}
Media: Video
import Image from 'next/image'
import Lightbox from '@/components/lightbox'
export default function LightboxVideoDemo() {
return (
<Lightbox
href="https://www.youtube.com/watch?v=Z1xX1Kt9NkU"
gallery="video"
title="Video caption"
description="Description goes here."
className="hover-effect-opacity d-flex rounded-4 overflow-hidden"
style={{ maxWidth: 480 }}
>
<div className="position-absolute d-flex flex-column align-items-center top-0 start-0 w-100 h-100 z-2 text-white p-4">
<span className="btn btn-icon btn-lg position-absolute top-50 translate-middle-y bg-white text-dark rounded-circle">
<i className="ci-play-filled"/>
</span>
<span className="hover-effect-target fs-sm fw-medium text-center opacity-0 mt-auto">Video caption</span>
</div>
<span className="hover-effect-target position-absolute top-0 start-0 w-100 h-100 bg-black bg-opacity-25 opacity-0 z-1"></span>
<Image src="/img/blog/grid/v2/video02.jpg" width={602} height={422} alt="Image" />
</Lightbox>
)
}
Media: Iframe (Google map)
import Image from 'next/image'
import OverlayTrigger from 'react-bootstrap/OverlayTrigger'
import Popover from 'react-bootstrap/Popover'
import PopoverBody from 'react-bootstrap/PopoverBody'
import Lightbox from '@/components/lightbox'
export default function LightboxIframeDemo() {
return (
<div className="position-relative bg-body-tertiary rounded-4 overflow-hidden">
<OverlayTrigger
placement="top"
overlay={
<Popover>
<PopoverBody>Click to view the map</PopoverBody>
</Popover>
}
>
<Lightbox
href="https://www.google.com/maps/embed?pb=!1m18!1m12!1m3!1d30908.594922615324!2d-73.07331970206108!3d40.788157341303005!2m3!1f0!2f0!3f0!3m2!1i1024!2i768!4f13.1!3m3!1m2!1s0x89e8483b8bffed93%3A0x53467ceb834b7397!2s396%20Lillian%20Blvd%2C%20Holbrook%2C%20NY%2011741%2C%20USA!5e0!3m2!1sen!2s!4v1706086459668!5m2!1sen!2"
gallery="map"
fullscreen
className="position-absolute top-50 start-50 translate-middle z-2 mt-lg-n3"
style={{ width: 50 }}
aria-label="Toggle map"
>
<svg xmlns="http://www.w3.org/2000/svg" viewBox="0 0 42.5 54.6">
<path
d="M42.5 19.2C42.5 8.1 33.2-.7 22 0 12.4.7 4.7 8.5 4.2 18c-.2 2.7.3 5.3 1.1 7.7h0s3.4 10.4 17.4 25c.4.4 1 .4 1.4 0 13.6-13.3 17.4-25 17.4-25h0c.6-2 1-4.2 1-6.5z"
fill="#ffffff"
/>
<g fill="#222934">
<path d="M20.4 31.8c-4.5 0-8.1-3.6-8.1-8.1s3.6-8.1 8.1-8.1 8.1 3.6 8.1 8.1-3.7 8.1-8.1 8.1zm0-14.2a6.06 6.06 0 0 0-6.1 6.1 6.06 6.06 0 0 0 6.1 6.1c3.3 0 6.1-2.7 6.1-6.1s-2.8-6.1-6.1-6.1z" />
<circle cx="20.4" cy="23.7" r="3" />
<path d="M20.4 54.5c-.6 0-1.1-.2-1.4-.6C5 39.3 1.5 29 1.4 28.5a21.92 21.92 0 0 1-1.2-8c.6-10.1 8.6-18.3 18.7-19C24.6 1.1 30 3 34.1 6.9c4.1 3.8 6.4 9.2 6.4 14.8 0 2.4-.4 4.7-1.2 6.9-.1.5-4 12-17.6 25.3-.3.4-.8.6-1.3.6zm-17-26.2c.8 2 4.9 11.6 17 24.2 13.2-13 17-24.5 17.1-24.6.7-2 1.1-4.1 1.1-6.3 0-5-2.1-9.9-5.8-13.3-3.7-3.5-8.6-5.2-13.7-4.8-9.1.6-16.4 8-16.9 17.1-.1 2.5.2 5 1.1 7.3l.1.4z" />
</g>
</svg>
</Lightbox>
</OverlayTrigger>
<Image src="/img/contact/map.jpg" fill sizes="1920px" className="object-fit-cover" alt="Map" />
{/* Add "ratio" element to avoid content shifts on page load */}
<div className="ratio ratio-16x9 d-none d-sm-block" />
<div className="ratio ratio-4x3 d-sm-none" />
<span className="position-absolute top-0 start-0 z-1 w-100 h-100 bg-body opacity-25"></span>
</div>
)
}
Zoom effect gallery
import Image from 'next/image'
import Row from 'react-bootstrap/Row'
import Col from 'react-bootstrap/Col'
import Lightbox from '@/components/lightbox'
export default function LightboxZoomGalleryDemo() {
return (
<Row xs={2} sm={3} className="g-3 g-xl-4">
{[
'/img/shop/fashion/product/02.png',
'/img/shop/fashion/product/04.png',
'/img/shop/fashion/product/05.png',
].map((image, index) => (
<Col key={index}>
<Lightbox
href={image}
gallery="image-gallery"
className="hover-effect-scale hover-effect-opacity position-relative d-flex rounded overflow-hidden"
>
<i className="ci-zoom-in hover-effect-target fs-3 text-white position-absolute top-50 start-50 translate-middle opacity-0 z-2"/>
<Image
src={image}
width={306}
height={342}
className="hover-effect-target bg-body-tertiary rounded"
alt="Image"
/>
</Lightbox>
</Col>
))}
</Row>
)
}
Inside card
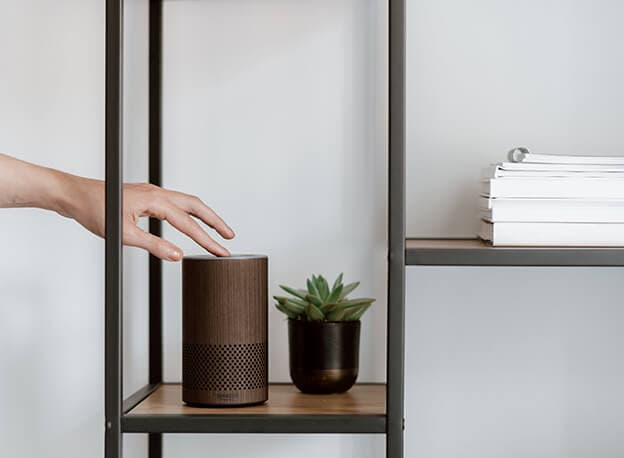
Card title
Some quick example text to build on the card title and make up the bulk of the card's content.
Go somewhereimport Image from 'next/image'
import Card from 'react-bootstrap/Card'
import CardBody from 'react-bootstrap/CardBody'
import CardTitle from 'react-bootstrap/CardTitle'
import CardText from 'react-bootstrap/CardText'
import Button from 'react-bootstrap/Button'
import Lightbox from '@/components/lightbox'
export default function LightboxCardDemo() {
return (
<Card style={{ maxWidth: 350 }}>
<Lightbox
href="/img/blog/grid/v1/07.jpg"
gallery="card"
className="hover-effect-scale hover-effect-opacity card-img-top d-flex position-relative overflow-hidden"
>
<i className="ci-zoom-in fs-2 position-absolute top-50 start-50 translate-middle text-white opacity-0 z-2"/>
<span className="hover-effect-target position-absolute top-0 start-0 w-100 h-100 bg-black bg-opacity-25 opacity-0 z-1"></span>
<Image src="/img/blog/grid/v1/07.jpg" width={416} height={305} alt="Card image" />
</Lightbox>
<CardBody>
<CardTitle as="h5">Card title</CardTitle>
<CardText>
Some quick example text to build on the card title and make up the bulk of the card's content.
</CardText>
<Button href="#">Go somewhere</Button>
</CardBody>
</Card>
)
}