Reviews
Display customer feedback with ratings, enhancing credibility and informing potential buyers.
Product review: Variant 1
Andrew Richards
May 7, 2025- Color: Purple
- Model: 128GB
The iPhone 14 is nothing short of exceptional, combining cutting-edge technology with a design that continues to set the standard for premium smartphones. That's why it deserves a perfect 5-star rating.
- Pros: Unparalleled performance, great camera, stunning design
- Cons: No in my opinion
ReplyCartzilla CompanyMay 8, 2025
Thank you for your feedback! We are glad that you were satisfied with your purchase :)
'use client'
import { useState } from 'react'
import ReviewVariantOne from '@/components/reviews/review-variant-one'
import Badge from 'react-bootstrap/Badge'
export default function ReviewsProductVariantOneDemo() {
const [likeCount, setLikeCount] = useState(29)
const [dislikeCount, setDislikeCount] = useState(5)
const [userLike, setUserLike] = useState(false)
const [userDislike, setUserDislike] = useState(false)
const handleLike = () => {
if (userLike) {
setLikeCount(likeCount - 1)
setUserLike(false)
} else {
setLikeCount(likeCount + 1)
if (userDislike) {
setDislikeCount(dislikeCount - 1)
setUserDislike(false)
}
setUserLike(true)
}
}
const handleDislike = () => {
if (userDislike) {
setDislikeCount(dislikeCount - 1)
setUserDislike(false)
} else {
setDislikeCount(dislikeCount + 1)
if (userLike) {
setLikeCount(likeCount - 1)
setUserLike(false)
}
setUserDislike(true)
}
}
return (
<ReviewVariantOne
author="Andrew Richards"
verified // Can also be a string of text that is displayed in a tooltip
rating={5}
// darkRating // Switch to dark color (rating stars)
date="May 7, 2025"
product={{
Color: 'Purple',
Model: '128GB',
// Add as many Key: value pairs as you need
}}
text="The iPhone 14 is nothing short of exceptional, combining cutting-edge technology with a design that continues to set the standard for premium smartphones. That's why it deserves a perfect 5-star rating."
pros={{
// label: '' // default "Pros" label can be altered
list: ['Unparalleled performance', 'great camera', 'stunning design'],
}}
cons={{
// label: '' // default "Cons" label can be altered
list: ['No in my opinion'],
}}
replyButton={{
// label: '' // default "Reply" label can be altered
onClick: () => alert('Reply button clicked!'),
}}
likeButton={{
count: likeCount,
onClick: handleLike,
}}
dislikeButton={{
count: dislikeCount,
onClick: handleDislike,
}}
>
<div className="ps-3 pb-2">
<div className="d-flex align-items-center pt-3 pb-2 mb-1">
<Badge bg="primary" className="me-2">
Reply
</Badge>
<span className="h6 mb-0 me-4">Cartzilla Company</span>
<span className="text-body-secondary fs-sm">May 8, 2025</span>
</div>
<p className="fs-sm mb-0">
Thank you for your feedback! We are glad that you were satisfied with your purchase :)
</p>
</div>
</ReviewVariantOne>
)
}
Product review: Variant 2
Bessie Cooper
April 8, 2025
While the design of the chair is nice and it does add a touch of retro vibe to my space, I found the quality to be lacking. After just a few weeks of use, one of the legs started to wobble, and the seat isn't as comfortable as I had hoped. Disappointed with the durability. Additionally, the assembly process was a bit frustrating as some of the screws didn't align properly with the holes, requiring extra effort to secure them in place. Overall, while it looks good, I expected better quality for the price.
No, I don't recommend this product
Helpful?
'use client'
import { useState } from 'react'
import ReviewVariantTwo from '@/components/reviews/review-variant-two'
export default function ReviewsProductVariantTwoDemo() {
const [likeCount, setLikeCount] = useState(3)
const [userLike, setUserLike] = useState(false)
const handleLike = () => {
if (userLike) {
setLikeCount(likeCount - 1)
setUserLike(false)
} else {
setLikeCount(likeCount + 1)
setUserLike(true)
}
}
return (
<ReviewVariantTwo
author="Bessie Cooper"
verified // Can also be a string of text that is displayed in a tooltip
rating={2}
darkRating
date="April 8, 2025"
recommendation={['no']} // 'yes' | 'no'. Optionally you add the second string to override the text.
likeButton={{
// label: '' // default "Helpful?" label can be altered
count: likeCount,
onClick: handleLike,
}}
className="border-top border-bottom py-4"
>
While the design of the chair is nice and it does add a touch of retro vibe to my space, I found the quality
to be lacking. After just a few weeks of use, one of the legs started to wobble, and the seat isn't as
comfortable as I had hoped. Disappointed with the durability. Additionally, the assembly process was a bit
frustrating as some of the screws didn't align properly with the holes, requiring extra effort to secure them
in place. Overall, while it looks good, I expected better quality for the price.
</ReviewVariantTwo>
)
}
Inside card: Variant 1
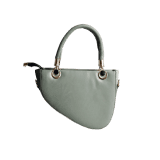
Alexandra D.
A wonderful compact bag, holds a lot of things, good tailoring, smooth seams, strong fittings, good quality.
import Image from 'next/image'
import Card from 'react-bootstrap/Card'
import StarRating from '@/components/reviews/star-rating'
export default function ReviewsCardVariantOneDemo() {
return (
<Card body className="rounded-4 p-sm-2" style={{ maxWidth: 416 }}>
<div className="d-flex align-items-center mb-3">
<Image
src="/img/home/fashion/v1/reviews/02.png"
className="flex-shrink-0"
width={160}
height={160}
alt="Image"
/>
<div className="ps-2 ms-1">
<StarRating rating={5} className="fs-xs pb-2 mb-1" />
<h3 className="h6 mb-0">Alexandra D.</h3>
</div>
</div>
<p className="mb-0">
A wonderful compact bag, holds a lot of things, good tailoring, smooth seams, strong fittings, good quality.
</p>
</Card>
)
}
Inside card: Variant 2
Perfect for daily use!
I bought the metallic blue bottle, and it has quickly become my go-to for everything! It keeps my water cold all day, even during my long shifts at work. Absolutely love it 🤩
import Image from 'next/image'
import Card from 'react-bootstrap/Card'
import CardBody from 'react-bootstrap/CardBody'
import CardFooter from 'react-bootstrap/CardFooter'
import StarRating from '@/components/reviews/star-rating'
export default function ReviewsCardVariantTwoDemo() {
return (
<Card className="bg-transparent border-0 rounded-5 shadow overflow-hidden p-xl-2" style={{ maxWidth: 416 }}>
<CardBody className="position-relative z-1 pb-1 pb-lg-2 pb-xl-3">
<StarRating rating={5} className="mb-3" />
<h3 className="h5 pb-2 mb-1">Perfect for daily use!</h3>
<p className="mb-0">
I bought the metallic blue bottle, and it has quickly become my go-to for everything! It keeps my water
cold all day, even during my long shifts at work. Absolutely love it 🤩
</p>
</CardBody>
<CardFooter className="position-relative z-1 d-flex align-items-center bg-transparent border-0 py-4">
<Image
src="/img/home/single-product/reviews/ava01.jpg"
className="flex-shrink-0 bg-body-secondary rounded-circle"
width={44}
height={44}
alt="Avatar"
/>
<div className="fs-sm ps-2 ms-1">
<div className="fw-semibold text-dark-emphasis">Jenny Wilson</div>
<div>Freshness Bottle</div>
</div>
</CardFooter>
<span className="position-absolute top-0 start-0 w-100 h-100 bg-white d-none-dark"></span>
<span
className="position-absolute top-0 start-0 w-100 h-100 bg-white d-none d-block-dark"
style={{ opacity: 0.08 }}
></span>
</Card>
)
}
Comment

Daniel Adams
August 15, 2025
I've used a lot of mockups, but these are some of the best. The variety of angles and perspectives really helped me showcase my app from different viewpoints. Plus, the customizable backgrounds are a fantastic feature — I could easily match the mockups to my brand colors. A must-have for designers!
'use client'
import { useState } from 'react'
import Comment from '@/components/reviews/comment'
export default function CommentDemo() {
const [likeCount, setLikeCount] = useState(6)
const [userLike, setUserLike] = useState(false)
const handleLike = () => {
if (userLike) {
setLikeCount(likeCount - 1)
setUserLike(false)
} else {
setLikeCount(likeCount + 1)
setUserLike(true)
}
}
return (
<Comment
author={{
avatar: '/img/shop/marketplace/single/comments/02.jpg',
name: 'Daniel Adams',
}}
date="August 15, 2025"
likeButton={{
count: likeCount,
active: userLike,
// label: '' // default "Like" aria-label can be altered
// className: '', // optionally apply extra CSS class to "Like" button
onClick: handleLike,
}}
replyButton={{
// label: '' // default "Reply" aria-label can be altered
// className: '', // optionally apply extra CSS class to "Reply" button
onClick: () => console.log('Reply button is clicked!'),
}}
>
I've used a lot of mockups, but these are some of the best. The variety of angles and perspectives really
helped me showcase my app from different viewpoints. Plus, the customizable backgrounds are a fantastic
feature — I could easily match the mockups to my brand colors. A must-have for designers!
</Comment>
)
}
Rating breakdown
4.2
68 reviews
5
37
4
16
3
8
2
4
1
3
import Row from 'react-bootstrap/Row'
import Col from 'react-bootstrap/Col'
import ProgressBar from 'react-bootstrap/ProgressBar'
import Stack from 'react-bootstrap/Stack'
import StarRating from '@/components/reviews/star-rating'
export default function ReviewsRatingBreakdownDemo() {
const ratings = [
{ ratingNumber: 5, ratingLabel: 'Five', quantity: 37 },
{ ratingNumber: 4, ratingLabel: 'Four', quantity: 16 },
{ ratingNumber: 3, ratingLabel: 'Three', quantity: 8 },
{ ratingNumber: 2, ratingLabel: 'Two', quantity: 4 },
{ ratingNumber: 1, ratingLabel: 'One', quantity: 3 },
]
const totalQuantity = ratings.reduce((sum, { quantity }) => sum + quantity, 0)
const weightedSum = ratings.reduce((sum, { ratingNumber, quantity }) => sum + ratingNumber * quantity, 0)
const averageRating = (weightedSum / totalQuantity).toFixed(1)
return (
<Row className="g-4">
<Col sm={5} md={3}>
<div className="d-flex flex-column align-items-center justify-content-center h-100 bg-body-tertiary rounded p-4">
<div className="h1 pb-2 mb-1">{averageRating}</div>
<StarRating rating={averageRating} className="fs-sm mb-2" />
<div className="fs-sm">{totalQuantity} reviews</div>
</div>
</Col>
<Col sm={7} md={9}>
<Stack direction="vertical" gap={3}>
{ratings.map(({ ratingNumber, ratingLabel, quantity }) => (
<Stack key={ratingNumber} direction="horizontal" gap={2} className="fs-sm">
<Stack direction="horizontal" gap={1}>
{ratingNumber}
<i className="ci-star-filled text-warning"/>
</Stack>
<ProgressBar
variant="warning"
now={(quantity / totalQuantity) * 100}
className="w-100"
style={{ height: 4 }}
aria-label={`${ratingLabel} stars`}
/>
<div className="text-nowrap text-end" style={{ width: 40 }}>
{quantity}
</div>
</Stack>
))}
</Stack>
</Col>
</Row>
)
}