Shop categories
Provide a navigational structure with links to different product categories for easy access and exploration.
Category card: Variant 1
import CategoryCardVariantOne from '@/components/shop/category-card-variant-one'
export default function CategoryCardVariantOneDemo() {
return (
<CategoryCardVariantOne
image={{
src: '/img/shop/electronics/categories/04.png',
width: 362,
height: 258,
// bg: 'none', // 'body' | 'body-tertiary' (default) | 'body-secondary' | 'primary' | 'secondary' | 'success' | 'danger' | 'warning' | 'info' | 'light' | 'dark' | 'white' | 'black' | 'primary-subtle' | 'success-subtle' | 'danger-subtle' | 'warning-subtle' | 'info-subtle'
// alt: 'Wearable Electronics', // Optionally set a custom image alt attribute, by default it is equal to title.
// style: { backgroundColor: '#e0e5eb' }, // Sets inline styles, like backgroundColor for more customization
}}
title="Wearable Electronics"
href="#"
list={[
{ label: 'Smart Watches', href: '#' },
{ label: 'Fitness Trackers', href: '#' },
{ label: 'Smart Glasses', href: '#' },
{ label: 'Clips, Arm & Wristbands', href: '#' },
{ label: 'Voice Recorders', href: '#' },
]}
style={{ maxWidth: 306 }}
/>
)
}
Category card: Variant 2
For men
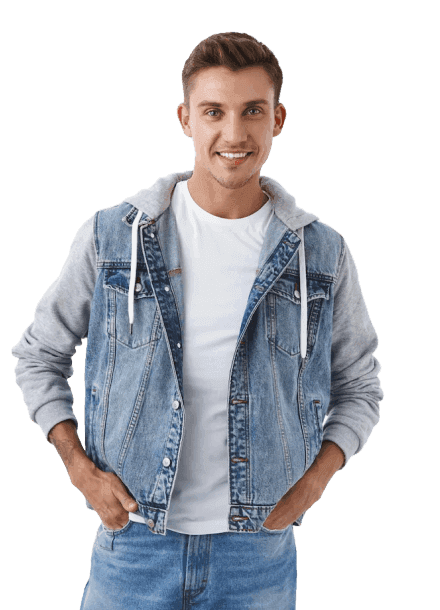
import CategoryCardVariantTwo from '@/components/shop/category-card-variant-two'
export default function CategoryCardVariantTwoDemo() {
return (
<CategoryCardVariantTwo
image={{
src: '/img/home/fashion/v2/categories/01.png',
// alt: 'For men', // Optionally set a custom image alt attribute, by default it is equal to title.
}}
title="For men"
href="#"
// buttonLabel="Shop now" // Optionally set a custom button label
list={[
{ label: 'Sports suits', href: '#' },
{ label: 'Trousers', href: '#' },
{ label: 'Jackets and coats', href: '#' },
{ label: 'Shirts', href: '#' },
]}
style={{ maxWidth: 416 }}
>
{/* Custom gradient background */}
<span
className="position-absolute top-0 start-0 w-100 h-100 d-none-dark"
style={{ background: 'linear-gradient(124deg, #e2daec -29.7%, #e4eefd 65.5%)' }}
></span>
<span
className="position-absolute top-0 start-0 w-100 h-100 d-none d-block-dark"
style={{ background: 'linear-gradient(124deg, #37343b -29.7%, #222a36 65.5%)' }}
></span>
</CategoryCardVariantTwo>
)
}
Category card: Variant 3
124 products
Only fresh fish to your table
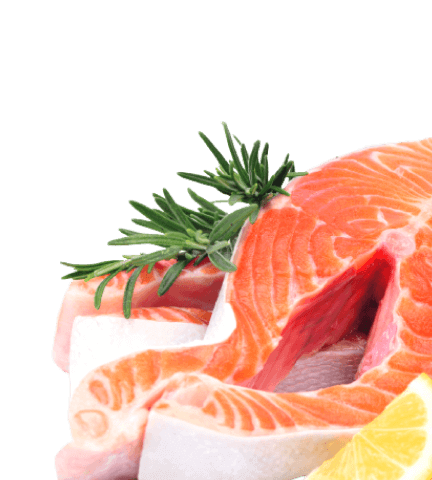
import CategoryCardVariantThree from '@/components/shop/category-card-variant-three'
export default function CategoryCardVariantThreeDemo() {
return (
<CategoryCardVariantThree
image={{
src: '/img/home/grocery/featured/01.png',
// alt: 'Image', // Optionally set a custom image alt attribute, by default it is equal to title.
}}
title="Only fresh fish to your table"
href="#"
eyebrowText="124 products"
// buttonLabel="Shop now" // Optionally set a custom button label
className="bg-primary-subtle"
style={{ maxWidth: 416 }}
/>
)
}
Category card: Variant 4
import CategoryCardVariantFour from '@/components/shop/category-card-variant-four'
export default function CategoryCardVariantFourDemo() {
return (
<CategoryCardVariantFour
image={{
src: '/img/home/furniture/categories/02.png',
// alt: 'Image', // Optionally set a custom image alt attribute, by default it is equal to title.
// square // Optionally make image square instead of circle
// bg: 'none', // 'body' | 'body-tertiary' (default) | 'body-secondary' | 'primary' | 'secondary' | 'success' | 'danger' | 'warning' | 'info' | 'light' | 'dark' | 'white' | 'black' | 'primary-subtle' | 'success-subtle' | 'danger-subtle' | 'warning-subtle' | 'info-subtle'
// alt: 'Living room', // Optionally set a custom image alt attribute, by default it is equal to title.
// style: { backgroundColor: '#e0e5eb' }, // Sets inline styles, like backgroundColor for more customization
}}
title="Living room"
href="#"
list={[
{ label: 'Bookcases and storage', href: '#' },
{ label: 'Coffee tables', href: '#' },
{ label: 'Cabinets', href: '#' },
]}
style={{ maxWidth: 216 }}
/>
)
}
Category card: Variant 5
import CategoryCardVariantFive from '@/components/shop/category-card-variant-five'
export default function CategoryCardVariantFiveDemo() {
return (
<CategoryCardVariantFive
image={{
src: '/img/home/marketplace/categories/03.jpg',
width: 268,
height: 178,
// alt: 'Image', // Optionally set a custom image alt attribute, by default it is equal to title.
}}
title="3D illustrations"
href="#"
style={{ maxWidth: 196 }}
/>
)
}
Category card: Variant 6
'use client'
import CategoryCardVariantSix from '@/components/shop/category-card-variant-six'
export default function CategoryCardVariantSixDemo() {
return (
<CategoryCardVariantSix
images={[
['/img/home/marketplace/collections/07.jpg', 'Image'],
['/img/home/marketplace/collections/08.jpg', 'Image'],
['/img/home/marketplace/collections/09.jpg', 'Image'],
]}
href="#"
title="Device mockups"
subtitle="305 resources"
// wishlistButton={false} // Disable Add to Wishlist button
wishlistButton={{
// active: true, // The wishlist button appears in an active state, indicating that the item has been added to the wishlist.
// labelAdd: 'Add to Wishlist', // Optionally override the button's aria-label in "add" state.
// labelRemove: 'Remove from Wishlist', // Optionally override the button's aria-label in "remove" state.
onClick: () => console.log('"Add to Wishlist" button is clicked!'),
}}
style={{ maxWidth: 306 }}
/>
)
}
Categories list
import Stack from 'react-bootstrap/Stack'
import CategoryList from '@/components/shop/category-list'
export default function CategoriesListDemo() {
return (
<Stack className="align-items-start gap-3 gap-sm-4">
{[
{
image: '/img/shop/grocery/categories/01.png',
title: 'Bakery & bread',
subtitle: '230 products',
href: '#',
},
{
image: '/img/shop/grocery/categories/02.png',
title: 'Vegetables',
subtitle: '180 products',
href: '#',
},
{
image: '/img/shop/grocery/categories/03.png',
title: 'Fresh fruits',
subtitle: '205 products',
href: '#',
},
].map(({ image, title, subtitle, href }, index) => (
<CategoryList
key={index}
href={href}
image={{
src: image
// alt: 'Image', // Optionally set a custom image alt attribute, by default it is equal to title.
// square // Optionally make image square instead of circle
// bg: 'none', // 'body' | 'body-tertiary' (default) | 'body-secondary' | 'primary' | 'secondary' | 'success' | 'danger' | 'warning' | 'info' | 'light' | 'dark' | 'white' | 'black' | 'primary-subtle' | 'success-subtle' | 'danger-subtle' | 'warning-subtle' | 'info-subtle'
// alt: 'Category image', // Optionally set a custom image alt attribute, by default it is equal to title.
// style: { backgroundColor: '#e0e5eb' }, // Sets inline styles, like backgroundColor for more customization
}}
title={title}
subtitle={subtitle}
/>
))}
</Stack>
)
}